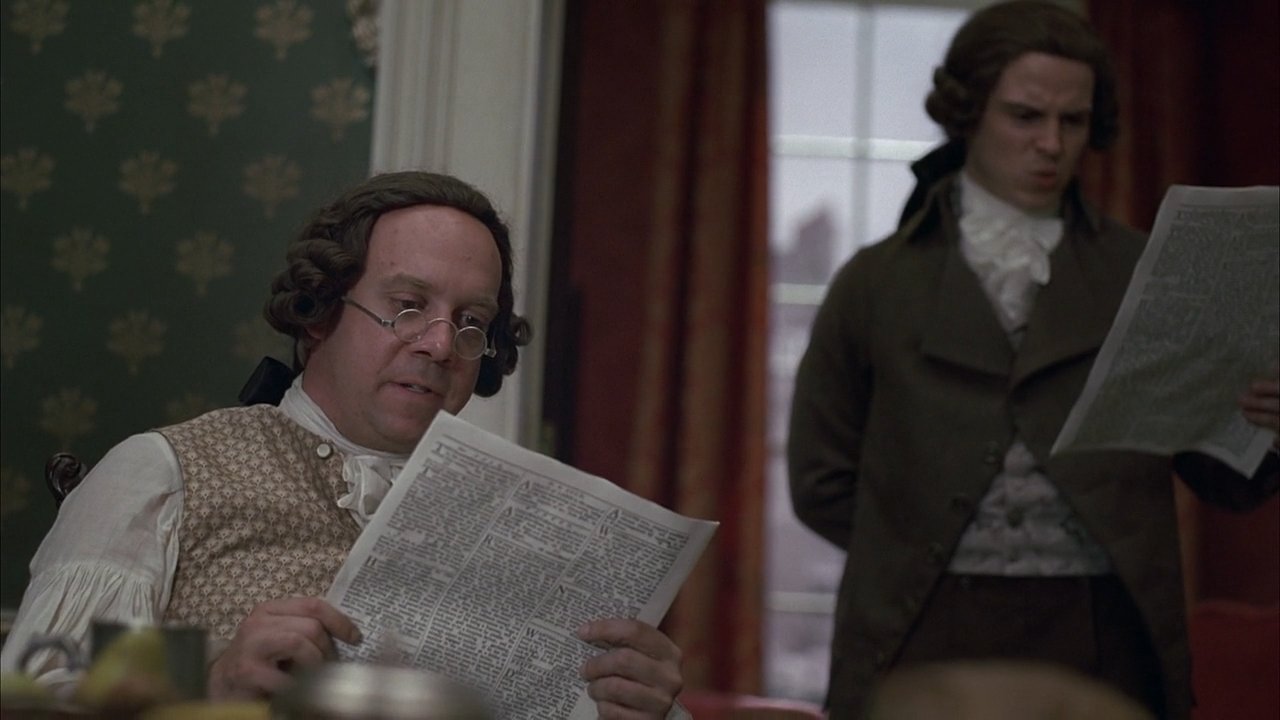
Paul Ford, in his great essay The Web Is a Customer Service Medium:
“Why wasn’t I consulted,” which I abbreviate as WWIC, is the fundamental question of the web. It is the rule from which other rules are derived. Humans have a fundamental need to be consulted, engaged, to exercise their knowledge (and thus power), and no other medium that came before has been able to tap into that as effectively.
Yet even with things like Wikipedia as an outlet, people still feel outraged when they aren’t consulted about something. It’s an unsolvable problem. It’s like trying to eradicate fear; sure you can focus really hard on it, but it’s probably healthier to just realize you’ll be scared sometimes and that’s okay.
I see this WWIC outrage all the time. I especially see this at work. Like all the time.
Then there’s the variant: “Nobody asked me!” which is best invoked as a defense when someone else made a decision without you. Maybe it was a bad decision and people think you should’ve been there to stop it from being made. “Hey, they made that decision without me. I wasn’t involved. I wish they would’ve called me in because I really could’ve fixed everyone’s problems and saved the world. But alas. I had nothing to do with it. Therefore, I’m not really emotionally invested in helping with the fallout either.”
While this victim-card-playing character I’ve painted here is a little exaggerated (but not much), they seriously could’ve actually been able to prevent a fallout from a bad decision!
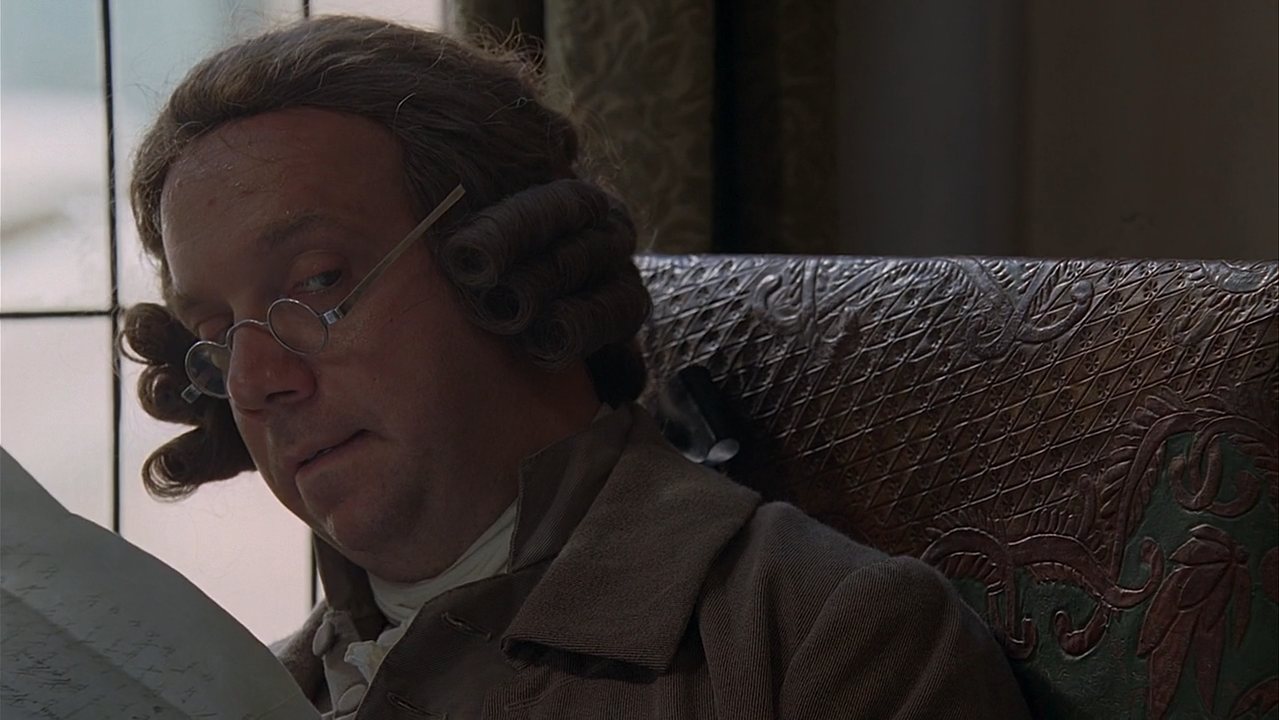
So it’s worth considering: why weren’t you consulted?
I’ve thought a lot about this myself. And these are the reasons I came up with. In your scenario, it’s probably a combination of them.
They didn’t even consider you.
They probably just forgot. I guarantee that most of the time it will be because they forgot about you. You just didn’t cross their mind as someone to engage.
Okay, so why is that?
They don’t know you.
Maybe literally like you’ve never met. Or maybe like they see you around but can never remember your name.
You lack the expertise.
You probably don’t lack the expertise. Otherwise you wouldn’t feel as outraged as you do about not being consulted. That said, consider this might be the case all the same.
You lack the context.
You may have the expertise. You might not have the context, though. And maybe the time it would take to give you the context isn’t worth the perceived value your consulting would deliver.
You lack the authority.
This certainly isn’t a prerequisite for being consulted. But being the boss awards you certain things—like being involved in decision making—and signing-off on decisions. If you have authority, perhaps you haven’t made it clear you expect sign-off. Or maybe you’re a little lacking in context or expertise. Remember, they probably just forgot to loop you in. Or…
You lack the bandwidth.
“We didn’t want to waste your time, and figured you had better things you could be doing anyway!” Consider that this is much more likely to happen if you feign busyness or make a show of how much unrelated responsibility you’ve otherwise accumulated. No problem should be too small for you engage with.
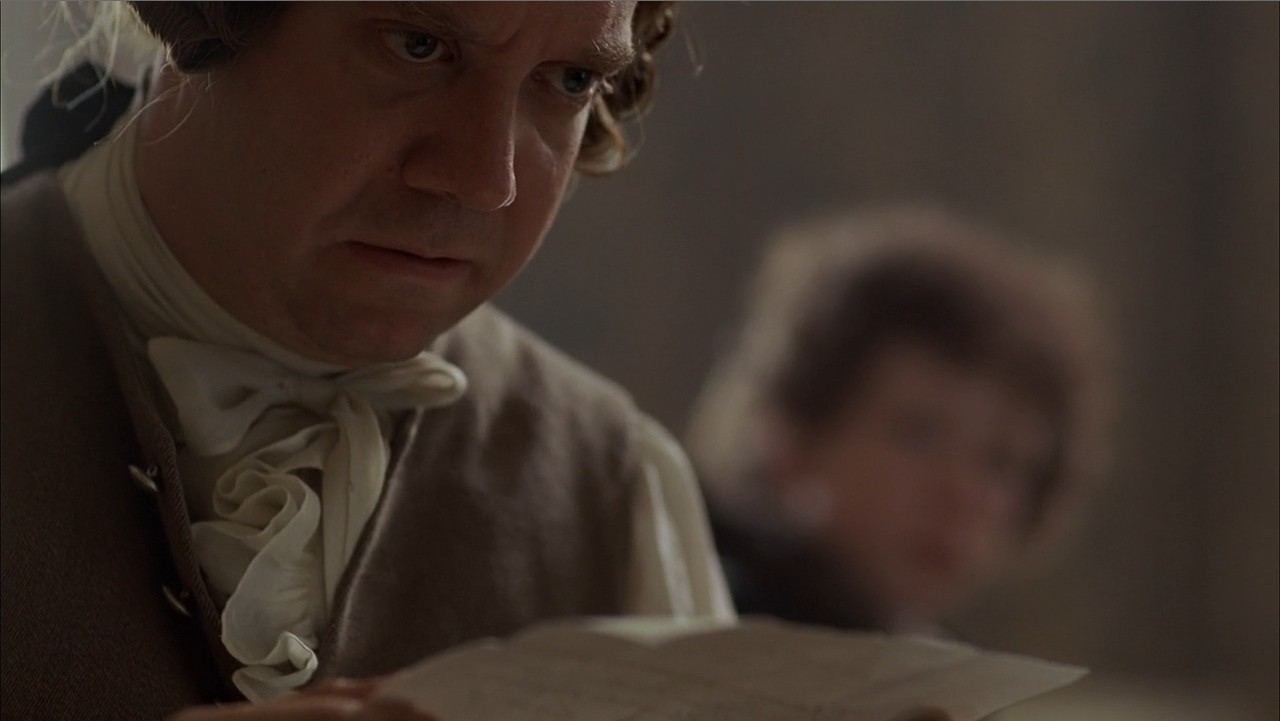
They don’t like you.
Ouch. A fancy way to say this might be “You lack the political capital” but that’s dressing-up the #realtalk. People like working with people they like. If you’re difficult, pretentious, overly verbose, or self-involved, people will avoid consulting you on anything, even if you have literally all of the above—expertise, context, and bandwidth.
A combination of these reasons are used when someone subconsciously decides who to engage.
You have to earn it.
This is all pointing to a common truth: the cost of including you outweighs the benefit in doing so.
One way to solve this problem in an organization is to bring down the cost of including people in a decision making process. For example, consider making decisions in a public place like a chat room that everyone has access to. You may be surprised at how well this scales. Plus, there’s a record of how the decision was made so you can later evaluate how a good of a decision was made. This probably only works if you have a blame free culture, though—and you probably don’t.
We’ve all been on both sides of this fence and constantly have the opportunity to see others raged over this stuff. It happens daily.
I wrote this for me. And you too. As a way to remember: it’s not a big deal. What you’re feeling is the result of a basic human need. Be aware of when you feel this. They probably just didn’t even think to ask you.
And that’s okay.